Use VCE Exam Simulator to open VCE files
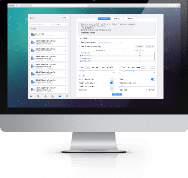
PCPP-32-101 Python Institute Practice Test Questions and Exam Dumps
Question 1
Which of the following statements accurately describes the concept of composition in object-oriented programming?
A. Composition extends a class’s capabilities by adding new components and modifying the existing ones
B. Composition allows a class to be projected as a container of different classes
C. Composition is a concept that promotes code reusability, while Inheritance promotes encapsulation
D. Composition is based on the has a relation, so it cannot be used together with inheritance
Correct answer: B
Explanation:
Understanding Composition:
In object-oriented programming, composition is a design principle where one class includes instances of other classes to achieve its functionality. This is often described as a "has-a" relationship. For example, a Car class might contain an Engine class, meaning a car has an engine.
This differs from inheritance, which is an "is-a" relationship. In inheritance, one class extends another (e.g., a Dog is an Animal). While inheritance allows a class to derive behavior and properties from a parent class, composition focuses on building complex types by combining objects of other types.
Why B is correct:
Option B is the most accurate representation of composition. It correctly implies that a class using composition can "contain" or be composed of other class instances. This allows greater flexibility, as you can change the behavior of a class by swapping out one of its components without modifying its overall structure.
Why the other options are incorrect:
A is misleading. While composition adds capabilities to a class by using other classes, it does not typically "modify" existing components—those components remain unchanged and are just used inside the new class.
C is incorrect because it misrepresents the benefits of composition and inheritance. Inheritance supports code reusability, not just encapsulation. Encapsulation refers to hiding internal state and requiring all interaction to be performed through methods. Composition also promotes code reusability through delegation.
D is incorrect because while composition is indeed based on the "has-a" relationship, it can be used together with inheritance. There's no rule that prevents combining them. For example, a class might inherit from a base class and also include other objects via composition to extend functionality.
Composition is a flexible design strategy that allows classes to incorporate functionality from multiple sources by containing instances of other classes.
Question 2
Given the Python code snippet, what is the most accurate statement about its behavior, particularly regarding exceptions?
A. The code is an example of implicitly chained exceptions.
B. The code is erroneous as the OwnMath class does not inherit from any Exception type class.
C. The code is fine and the script execution is not interrupted by any exception.
D. The code is an example of explicitly chained exceptions.
Correct answer: B
Explanation:
Understanding the Code Context:
Here is the relevant code:
class OwnMath:
pass
def calculate_value(numerator, denominator):
try:
value = numerator / denominator
except ZeroDivisionError as e:
raise OwnMath from e
return value
calculate_value(4, 0)
This function attempts to divide 4 by 0, which causes a ZeroDivisionError. The exception is caught in the except block, and a new exception OwnMath is raised using raise ... from e. This syntax is intended for exception chaining, specifically explicit exception chaining.
However, the key issue here is that OwnMath does not inherit from Python's built-in Exception or BaseException classes. In Python, only instances or subclasses of BaseException can be raised using raise. Since OwnMath is just a plain class with no inheritance, attempting to raise OwnMath from e will result in a TypeError, such as:
TypeError: exceptions must derive from BaseException
This makes the code erroneous.
Analysis of the Options:
A (Implicitly chained exceptions): Implicit chaining occurs when one exception is raised while handling another, without explicitly using from. That’s not what happens here.
B (Correct): Since OwnMath does not inherit from Exception or BaseException, it cannot be raised. This leads to a TypeError. Therefore, this is the correct answer.
C (Incorrect): The script execution is interrupted, and it fails with a TypeError. So, the script is not “fine.”
D (Incorrect): The intention is explicit chaining (raise ... from e), but because OwnMath is not a valid exception class, the syntax fails. This would be explicit chaining if the OwnMath class was correctly derived.
How to Fix the Code:
To make the code valid and allow exception chaining, OwnMath must inherit from Exception:
class OwnMath(Exception):
pass
With this change, the statement raise OwnMath from e would correctly raise an OwnMath exception, chained to the original ZeroDivisionError.
The code attempts explicit exception chaining but fails because OwnMath is not a subclass of Exception. This makes the code erroneous and leads to a runtime error.
Question 3
Given the Python class definition where var1 is defined at the class level and self.name is initialized in the constructor, which statement best describes the behavior of the variables in this code?
A. self.name is the name of a class variable
B. var1 is the name of a global variable
C. Excalibur is the value passed to an instance variable
D. weapon is the value passed to an instance variable
Correct answer: C
Explanation:
Understanding the Code:
class Sword:
var1 = 'weapon'
def __init__(self):
self.name = 'Excalibur'
There are two key types of variables in this class:
var1 is defined at the class level.
self.name is defined inside the constructor method __init__.
Let’s break them down:
var1 = 'weapon' is defined directly inside the class but outside of any instance method.
This makes it a class variable, which is shared among all instances of the class.
This is not a global variable. A global variable would be defined outside any class or function, in the main body of the script or module.
Therefore, option B is incorrect.
Inside the __init__ method, self.name = 'Excalibur' assigns the string 'Excalibur' to an instance variable named name.
This variable is unique to each object (or instance) created from the Sword class.
Since it is being assigned directly to self.name, 'Excalibur' is the value of the instance variable, not a passed argument, but an assigned one.
Thus, the correct interpretation is that Excalibur is the value given to an instance variable.
So, option C is correct.
A (self.name is the name of a class variable): Incorrect — self.name is an instance variable, not a class variable.
B (var1 is the name of a global variable): Incorrect — var1 is a class variable, not a global one.
C (Excalibur is the value passed to an instance variable): Correct — 'Excalibur' is assigned to an instance variable self.name.
D (weapon is the value passed to an instance variable): Incorrect — 'weapon' is the value assigned to a class variable, not an instance variable.
In Python, class variables are shared among all instances, while instance variables are specific to each instance. The value 'Excalibur' is directly assigned to an instance variable self.name, making it the correct and relevant detail.
Question 4
The code defines three separate classes, two of which contain a method named run(). A loop then attempts to call run() on instances of all three classes. Which OOP principle is best represented by this behavior?
A. Serialization
B. Inheritance
C. Encapsulation
D. Polymorphism
Correct answer: D
Explanation:
The code in the image consists of three classes, each defining different methods. Here's a simplified breakdown of the code snippet:
class A:
def run(self):
print("A is running")
class B:
def fly(self):
print("B is flying")
class C:
def run(self):
print("C is running")
for element in A(), B(), C():
element.run()
Let’s analyze what this code is doing, and which OOP concept it highlights.
Class A and Class C both define a method named run().
Class B does not define a run() method.
The for loop iterates over instances of A, B, and C and attempts to call element.run() on each.
This will lead to a AttributeError at runtime when it reaches the instance of class B, because B does not have a method named run().
However, despite the runtime error, this code is intended to demonstrate a key Object-Oriented Programming concept where different objects respond to the same method name (run) in their own way.
That concept is Polymorphism.
Polymorphism is one of the core pillars of OOP. It allows objects of different classes to be treated through the same interface. In this case, both A and C respond to run() even though they're separate classes.
The idea is: you can call run() on different types of objects, and each type can respond differently.
This is sometimes referred to as duck typing in Python — "If it walks like a duck and quacks like a duck..."
A. Serialization: This is the process of converting an object into a format that can be stored or transmitted. Irrelevant here.
B. Inheritance: No class is inheriting from another here, so inheritance is not demonstrated.
C. Encapsulation: Encapsulation involves hiding the internal state and requiring all interactions to be performed through methods. Not the focus of this example.
D. Polymorphism: Correct. The loop expects each object to respond to run(), which is polymorphic behavior. It shows that different objects can be treated similarly if they share a method name and signature.
This code demonstrates how different objects (instances of different classes) can be used interchangeably when they share a common method interface (run()), despite not inheriting from a common base class. This is classic polymorphism, specifically dynamic or runtime polymorphism.
Question 5
The code defines a decorator function that appears to take arguments and wraps another function in nested layers. Based on its structure, which statement best describes the code?
A. It is an example of a decorator that accepts its own arguments.
B. It is an example of decorator stacking.
C. It is an example of a decorator that can trigger an infinite recursion.
D. The function is erroneous.
Correct answer: A
Explanation:
To evaluate the correctness of this code and determine what it demonstrates, we need to understand Python decorators and how they can be parameterized.
Here is the simplified version of the code structure from the image:
def my_decorator(coating):
def level1_wrapper(my_function):
def level2_wrapper(*args):
my_function(*args)
return level2_wrapper
return level1_wrapper
This function structure defines a decorator factory — a function that returns a decorator, which in turn returns a wrapper function.
Let’s walk through it step by step:
def my_decorator(coating):
This function accepts a parameter named coating. This implies that the decorator is customizable — that is, we can decorate functions like:
@my_decorator('sugar')
def some_function():
...
So it’s not a standard decorator — it's a parameterized decorator.
def level1_wrapper(my_function):
This is the actual decorator returned by my_decorator(). It accepts the function to be decorated.
def level2_wrapper(*args):
my_function(*args)
This is the wrapper around the actual function, which forwards any arguments it receives to my_function. This is common in decorators that don’t know in advance how many arguments the decorated function will take.
level2_wrapper is returned by level1_wrapper.
level1_wrapper is returned by my_decorator.
This return chain enables the full three-layered structure needed for decorators that accept arguments.
B. Decorator stacking refers to applying multiple decorators to the same function using multiple @decorator_name lines. This is not shown here.
C. Infinite recursion would happen if the wrapper called itself directly or indirectly. Here, the wrapper calls the decorated function (my_function), not itself.
D. The function is not erroneous. All syntax and logic is sound.
The code is a valid, well-formed example of a decorator that accepts its own arguments. It involves a three-layer structure: the outermost function to capture arguments, a middle function to act as the decorator, and the innermost function to wrap the target function.
Question 6
Consider the function definition def f1(*arg, **args):. What is the most accurate statement about this code's correctness or behavior?
A. The code is syntactically correct despite the fact that the names of the function parameters do not follow the naming convention
B. The *arg parameter holds a list of unnamed parameters
C. The code is missing a placeholder for unnamed parameters
D. The code is syntactically incorrect - the function should be defined as def f1(*args, **kwargs)
Correct answer: D
Explanation:
This question tests your understanding of how variable-length arguments are handled in Python functions, specifically the use of *args and **kwargs.
*args is used to collect positional arguments as a tuple.
**kwargs is used to collect keyword arguments as a dictionary.
These names, args and kwargs, are simply conventions. You can use any valid variable name, such as *arg or **myparams, and the function will still be syntactically valid — as long as the parameter names are unique.
But here’s the issue:
def f1(*arg, **args):
pass
This function is not syntactically correct. The problem is that both the * and the ** arguments are using the same name root: arg and args. Although technically they are different variable names, Python treats them as overlapping in semantics, and it's ambiguous and not allowed.
More precisely:
The name args is already used as the name for **args, the keyword arguments.
Python requires that each parameter name in a function must be unique, and even though arg and args are different, they are too close semantically and can lead to confusion or even namespace issues in function internals.
Python will raise a SyntaxError for this definition.
A. Incorrect. The code is not syntactically correct. Even though names like arg or args are allowed, the function has a name collision problem, making it invalid.
B. Incorrect. While it is true that *arg would collect positional arguments, this does not justify the syntax issue present in the full function.
C. Incorrect. The function is not missing unnamed parameter placeholders; rather, it incorrectly defines them with a conflict.
D. Correct. The code is syntactically incorrect and should be properly written using standard conventions like def f1(*args, **kwargs):, where the names are distinct and non-conflicting.
The function fails due to a naming conflict between *arg and **args. Python requires that parameter names be unique, and this function violates that rule. The correct version would use something like *args and **kwargs.
Question 7
Given the Python class Crossword, determine whether the code is valid and identify which method(s), if any, should be declared as class methods using @classmethod.
A. There is only one initializer, so there is no need for a class method
B. The getNumberOfCrosswords() method should be decorated with @classmethod
C. The code is erroneous
D. The getNumberOfCrosswords() and isSolved() methods should be decorated with @classmethod
Correct answer: B
Explanation:
This question examines your understanding of class methods, static methods, and instance methods in Python.
Instance Method (default): Takes self as the first parameter. Accesses and modifies instance attributes.
Class Method: Takes cls as the first parameter. Accesses or modifies class attributes.
Static Method: No self or cls. Behaves like a regular function but lives in the class's namespace.
Let’s analyze the methods defined in the Crossword class.
This is the constructor, which initializes the instance attributes height, width, and progress. It’s correctly defined as an instance method and needs no modification.
This method is decorated as @staticmethod, meaning it should not use self or cls. But inside the method, we see:
if self.isSolved():
This is invalid. A static method cannot access self, because it doesn’t receive any instance reference. Therefore, this method is erroneous in its current form.
It either needs:
To be turned into an instance method by adding self as the first parameter
or
Avoid referring to self entirely and work without instance context
Thus, this static method is incorrectly implemented, but this doesn’t make the entire code structurally erroneous—it’s a logical error in usage.
This method uses self.progress, meaning it operates on instance-level data. It is properly defined as an instance method and should not be decorated with @classmethod.
So, Option D is incorrect.
This method takes cls as a parameter and accesses the class variable number_of_Crosswords.
This is the textbook use case for a class method, but it is missing the @classmethod decorator.
In Python, just naming the first parameter cls doesn't make a method a class method; you must explicitly use the @classmethod decorator. Otherwise, Python treats it as a regular instance method and passes an instance (not the class), resulting in potential attribute access errors.
So, this is an instance method that tries to behave like a class method, which is incorrect unless decorated properly.
A. Incorrect. This refers to the constructor and misses the core issue with getNumberOfCrosswords().
B. Correct. getNumberOfCrosswords() uses cls and accesses a class variable, so it should be decorated with @classmethod.
C. Incorrect. While isElementCorrect is misused as a static method, this alone doesn't make the entire class definition syntactically erroneous.
D. Incorrect. isSolved() is correctly defined as an instance method—it uses self appropriately and doesn’t need to be a class method.
The only required correction is to decorate getNumberOfCrosswords() with @classmethod since it accesses a class variable. The misuse of @staticmethod in isElementCorrect() is incorrect but doesn't make the code completely erroneous from a syntax standpoint—it’s a logical misuse.
Question 8
Examine the given Python class Item, which includes the special method __ne__, and determine which statement best explains its purpose or correctness.
A. __ne__() is not a built-in special method.
B. The code is erroneous
C. The code is responsible for the support of the negation operator, e.g. a = -a
D. The code is responsible for the support of the inequality operator, i.e. !=
Correct answer: D
Explanation:
This question tests your understanding of Python's special (magic) methods—specifically, those involved in operator overloading and object comparisons.
The class in the question is:
class Item:
def __init__(self, initial_value):
self.value = initial_value
def __ne__(self, other):
...
This class includes:
An __init__() constructor method to initialize the instance.
A __ne__() method, which is the special method Python uses to define behavior for the != operator.
Let’s analyze each option.
Incorrect.
This is false. The __ne__() method is a built-in Python special method. It is the counterpart to __eq__() and is used to define how objects respond to the != operator. If you do not define __ne__, Python will attempt to derive it from __eq__, but explicitly defining __ne__ allows for custom inequality behavior.
Incorrect.
The code is not syntactically or semantically incorrect. It defines a constructor and a special method. Even though the __ne__() method's body is shown as an ellipsis (...), which might indicate incomplete implementation, this is valid Python syntax (it’s just a placeholder for code). Therefore, there is no error here.
Incorrect.
The negation operator (-a) is handled by the __neg__() method, not __ne__(). This is a subtle but important distinction. The __neg__() method would be the correct one for overloading unary negation. Hence, this option misidentifies the purpose of __ne__().
Correct.
This is accurate. In Python, when the != operator is used to compare two objects, Python looks for the __ne__() method. If it's not defined, Python attempts to use not __eq__() as a fallback. However, when custom inequality logic is required, the __ne__() method should be explicitly implemented.
The presence of __ne__() in the Item class means that the class is being set up to support custom behavior when two objects of this class are compared using !=.
__ne__() is a built-in method.
The code is not erroneous.
__ne__() is not for unary negation—that’s __neg__().
__ne__() does handle the != operator.
Question 9
What function or operator should be used to determine whether two variables refer to the exact same object in memory, returning True or False?
A. The == operator
B. The isinstance() function
C. The id() function
D. The is function
Correct answer: D
Explanation:
In Python, there is a critical distinction between object identity and object equality. The question asks whether two variables refer to the same object, not whether they are equal in value.
Object identity means that two variables point to the same object in memory.
Object equality means that two objects may have the same contents, but are not necessarily the same object in memory.
Let’s evaluate each option in the context of checking object identity.
Incorrect.
The == operator checks whether the values of two variables are equal—not whether the variables point to the same object. You can override the __eq__() method in a class to define custom behavior for ==. Therefore, even if a == b evaluates to True, a is b may still be False.
Example:
a = [1, 2, 3]
b = [1, 2, 3]
print(a == b) # True - same values
print(a is b) # False - different objects in memory
Incorrect.
The isinstance() function is used to check if an object is an instance of a particular class or a subclass thereof. It doesn’t compare two objects directly at all, and it definitely does not determine if two variables point to the same object.
Example:
x = 5
print(isinstance(x, int)) # True
Incorrect (though partially related).
While the id() function returns the unique memory address of an object, comparing id(a) == id(b) will tell you if two variables refer to the same object. However, this isn't the most Pythonic or direct way to check object identity. It returns an integer, and the comparison of IDs is more error-prone than using is.
Example:
a = [1]
b = a
print(id(a) == id(b)) # True
This works but isn't idiomatic Python; using is is better.
Correct.
The is operator is explicitly designed to check whether two variables refer to the same object in memory. This is the most direct, readable, and reliable way to answer the question “Do two variables refer to the same object?”
Example:
a = [1]
b = a
c = [1]
print(a is b) # True
print(a is c) # False
To determine whether two variables point to the same object, you should use the is operator. It returns True if both variables reference the same memory location, and False otherwise.
Question 10
Which of the following statements about the @property decorator in Python is false?
A. The @property decorator should be defined after the method that is responsible for setting an encapsulated attribute.
B. The @property decorator designates a method which is responsible for returning an attribute value.
C. The @property decorator marks the method whose name will be used as the name of the instance attribute.
D. The @property decorator should be defined before the methods that are responsible for setting and deleting an encapsulated attribute.
Correct answer: A
Explanation:
The @property decorator in Python allows you to define a method that acts like an attribute. It's a powerful feature for encapsulating data access while keeping a clean syntax. Let’s examine each statement to determine which one is false.
False.
This statement is incorrect and thus is the correct answer to the question. In Python, the @property decorator is used before the method definition. Moreover, the setter method (defined with @<propertyname>.setter) must come after the getter method that is decorated with @property. This statement in Option A reverses the required order of declaration, so it is false.
Correct order:
class MyClass:
def __init__(self):
self._x = 0
@property
def x(self):
return self._x
@x.setter
def x(self, value):
self._x = value
True.
This is a correct description. When you use @property, the decorated method becomes a getter that returns a value like a regular attribute.
True.
This is accurate. If you decorate a method called x with @property, then x becomes accessible as an attribute (e.g., obj.x). The method name becomes the property name.
True.
This is also correct. The getter must be defined first using @property, followed by the setter (@x.setter) and deleter (@x.deleter) methods.
Top Training Courses
LIMITED OFFER: GET 30% Discount
This is ONE TIME OFFER
A confirmation link will be sent to this email address to verify your login. *We value your privacy. We will not rent or sell your email address.
Download Free Demo of VCE Exam Simulator
Experience Avanset VCE Exam Simulator for yourself.
Simply submit your e-mail address below to get started with our interactive software demo of your free trial.