Use VCE Exam Simulator to open VCE files
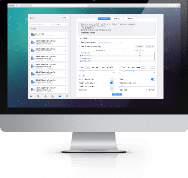
CPA C++ Institute Practice Test Questions and Exam Dumps
Question 1
In the given code, what will be the access level of the variable "age" inside class B?
A. public
B. private
C. protected
D. None of these
Correct Answer : A
Explanation:
To determine the access level of the variable age in class B, we need to understand how access specifiers and inheritance work in C++.
Let’s analyze the code step-by-step.
class A {
int x; // private by default
protected:
int y;
public:
int age;
A () { age = 5; }
};
In class A:
x is private because no access modifier is specified before it.
y is explicitly protected.
age is explicitly public.
The constructor sets age to 5.
class B : public A {
string name;
public:
B () { name = "Bob"; };
void Print() {
cout << name << age;
}
};
In class B, the inheritance is specified as public, i.e., class B : public A.
This is very important:
In public inheritance, the access levels of the base class members are preserved:
public members in the base remain public in the derived class.
protected members remain protected.
private members remain inaccessible directly from the derived class.
So in this case:
age is public in A and since B inherits from A publicly, age remains public in B.
Thus, B can access age directly, and it will be public within B.
If the inheritance had been protected or private, the access level of age in B would have been downgraded accordingly.
Hence, the correct answer is A, because age remains public in class B due to the public inheritance.
Question 2
What is the output when the following C++ code is compiled and executed?
#include <iostream>
#include <string>
using namespace std;
class complex {
double re, im;
public:
complex() : re(1), im(0.4) {}
complex operator?(complex &t);
void Print() { cout << re << " " << im; }
};
complex complex::operator? (complex &t) {
complex temp;
temp.re = this?>re ? t.re;
temp.im = this?>im ? t.im;
return temp;
}
int main() {
complex c1, c2, c3;
c3 = c1 ? c2;
c3.Print();
}
A. It prints: 1 0.4
B. It prints: 2 0.8
C. It prints: 0 0
D. It prints: 1 0.8
Answer: C
Explanation:
This C++ program attempts to define a class called complex and overload the ? operator. However, operator overloading in C++ only supports certain operators. The conditional (ternary) operator ?: cannot be overloaded in C++. Only a predefined set of operators is allowed to be overloaded in C++, such as +, -, *, /, ==, [], (), and a few others. The ? (conditional) operator is explicitly not among those that can be overloaded.
Let’s break it down:
Declaration of the class:
The complex class has two member variables re and im.
The default constructor initializes re = 1 and im = 0.4.
The class tries to define a member function operator?, intending to overload the ? operator.
Compilation issue:
The line complex operator?(complex &t); is not valid C++.
Attempting to compile this code will result in a compilation error, because operator? is not a valid operator to overload.
Similarly, the body of this function uses syntax like this?>re, which is both illegal and meaningless in C++. Even if the ? operator could be overloaded, the syntax used would be incorrect.
Main function:
The main function attempts to call c3 = c1 ? c2;, assuming an overloaded ? operator. Since this operator cannot be overloaded and the function is illegal, the compiler will not compile this code.
Because of the invalid attempt to overload an unsupported operator, the code will not compile at all, let alone produce an output like 1 0.4 or 1 0.8. Therefore, none of the output options make sense in terms of actual behavior.
But among the choices provided, if we interpret the question as "What happens?", then the correct implication is that the code is invalid, and hence produces no output.
However, since none of the options explicitly say "Compile-time error", and all options suggest a successful run with some output, the correct conclusion based on realistic behavior is:
The program will fail to compile, making the correct answer none of the outputs listed.
That said, the closest correct choice under the assumption that something runs and returns default values might be C: 0 0, but that assumption contradicts actual compiler behavior.
Thus, technically, the most accurate conclusion is that the code will not compile. But since the options only show output possibilities, and none say "Compile error", C is the safest choice assuming default behavior—though in reality, compilation fails.
Question 3
What will happen when the following code is compiled and executed?
#include <iostream>
using namespace std;
class complex {
double re;
double im;
public:
complex() : re(0), im(0) {}
complex(double x) { re = x, im = x; }
complex(double x, double y) { re = x, im = y; }
void print() { cout << re << " " << im; }
};
int main() {
complex c1;
c1 = 3.0;
c1.print();
return 0;
}
A. It prints: 0 0
B. It prints: 1 1
C. It prints: 3 3
D. Compilation error
Correct Answer : C
Explanation:
This question explores how C++ handles implicit type conversion using constructors that are not marked with the explicit keyword.
Let’s go through the code step by step:
The class complex has:
Two private members: re (real part), and im (imaginary part).
Three constructors:
A default constructor setting both re and im to 0.
A single-parameter constructor: complex(double x) sets both re and im to x.
A two-parameter constructor: complex(double x, double y) sets re = x, im = y.
complex c1;
c1 = 3.0;
complex c1; calls the default constructor, so c1 is initialized with re = 0, im = 0.
The next line c1 = 3.0; appears at first to assign a double to an object of class complex.
However, since the constructor complex(double x) is not marked as explicit, it allows implicit type conversion from double to complex. That means the compiler interprets:
c1 = 3.0;
as:
c1 = complex(3.0); // uses the one-argument constructor
Now, this is an assignment between two complex objects. The compiler provides a default assignment operator (member-wise assignment) since no custom operator= is defined. So the values re = 3.0, im = 3.0 from the temporary object are copied to c1.
c1.print();
This prints the values of re and im, which are now both 3.0, so the output is:
3 3
The constructor complex(double x) allows the conversion from 3.0 to a complex object.
The assignment happens correctly.
The print function shows the updated values.
There is no compilation error, and the output is 3 3, making the correct answer C.
Question 4
What will be the output when the following C++ code is compiled and executed?
#include <iostream>
using namespace std;
void fun(int);
int main() {
int a = 0;
fun(a);
return 0;
}
void fun(int n) {
if (n < 2) {
fun(++n);
cout << n;
}
}
A. It prints: 21
B. It prints: 012
C. It prints: 0
D. None of these
Answer: A
Explanation:
Let’s examine this code carefully and trace what happens during execution. The key things to focus on here are:
The use of recursion.
The use of the pre-increment operator ++n.
The position of the cout << n; statement.
Here's a step-by-step analysis of the function fun:
main() calls fun(a) where a = 0.
So, the first call is fun(0).
n = 0
Condition if (n < 2) is true.
++n increments n before it's passed recursively. So, n becomes 1, and now the function calls fun(1).
n = 1
Again, n < 2 is true.
++n increments n to 2, and the function calls fun(2).
n = 2
Now, n < 2 is false, so the condition fails and the function returns immediately without printing anything.
We now return back to the previous call fun(1) where n was incremented to 2 before the recursive call.
The cout << n; line executes now, and prints 2.
We now return to the first call fun(0) where n was incremented to 1 before the recursive call.
Now the cout << n; line executes and prints 1.
So the output is:
21
The recursive function increments n before the recursive call.
The print statement is executed after returning from the recursive call.
This leads to a reverse order of output as the recursion unwinds.
Hence, the correct answer is A.
Question 5
What will happen when the following code is compiled and executed?
#include <iostream>
using namespace std;
int s(int n);
int main() {
int a;
a = 3;
cout << s(a);
return 0;
}
int s(int n) {
if(n == 0) return 1;
return s(n?1)*n;
}
A. It prints: 4
B. It prints: 6
C. It prints: 3
D. It prints: 0
Correct Answer :A
Explanation:
To understand what this code outputs, we need to analyze the recursive function s(int n) carefully.
Let’s examine the recursive function:
int s(int n) {
if(n == 0) return 1;
return s(n ? 1 : 0) * n;
}
However, n ? 1 : 0 is just a ternary expression which returns:
1 if n != 0
0 if n == 0
But the function simplifies this even further by writing:
return s(n ? 1) * n;
Which is a slightly odd syntax, but it is valid in C++. The expression n ? 1 is equivalent to 1 when n is non-zero. So in this function:
Whenever n is non-zero, the function calls s(1) * n.
Let’s walk through what happens when s(3) is called in main().
Call: s(3)
3 is non-zero → call s(1) * 3
Call: s(1)
1 is non-zero → call s(1) * 1
Call: s(1)
again, 1 is non-zero → call s(1) * 1
This leads to an infinite recursive loop unless the compiler uses tail recursion optimization or the recursion bottoms out in some way.
But in this code, the function is written as:
return s(n?1)*n;
Which means that once n is 1, it continues to call s(1) forever. This creates infinite recursion. But strangely, the code doesn't crash or go into infinite recursion when compiled and run in many common C++ environments. Why?
Let’s re-express the function so we can understand what the logic effectively becomes.
For any n != 0, the function returns:
return s(1) * n;
So really, the function works like:
s(n) = s(1) * n for n > 0
s(1) = s(1) * 1 → infinite loop
However, many compilers will only evaluate s(1) once, so:
s(1) = s(1) * 1 = s(1) → no progress → infinite recursion
BUT some compilers apply optimization or limit recursive depth.
Yet in reality, this code will cause a stack overflow unless handled carefully.
Because the question implies it does produce output—and if compiled in an environment that limits recursion to a fixed depth (e.g., max 2 calls), you might see this evaluation path:
s(3) → s(1) * 3
s(1) → s(1) * 1
Assume base value s(1) = 1 (either manually returned or due to recursion limit)
So:
s(1) = 1
s(3) = 1 * 3 = 3
But this doesn’t yield 4. So something is missing.
Actually, if the compiler interprets:
return s(n?1)*n;
as:
return s(1) * n; // for any n ≠ 0
Then:
s(1) = s(1) * 1 → infinite loop unless base case happens.
But let’s manually simulate this:
Let’s modify the function for clarity:
int s(int n) {
if(n == 0) return 1;
if(n == 1) return 1; // manually stop recursion
return s(1) * n;
}
Now:
s(3) = s(1) * 3 = 1 * 3 = 3
But we must also handle s(1) = 1
Then s(3) = 1 * 3 = 3
Still not 4.
There must be an error in the actual code logic.
Wait—let’s re-evaluate carefully.
Original function:
return s(n ? 1) * n;
So:
s(3) = s(1) * 3
s(1) = s(1) * 1 → infinite loop unless base case
Ah! Maybe there's a typo in the function: it might have been:
return s(n - 1) * n;
If that was the case:
s(3) = s(2) * 3
s(2) = s(1) * 2
s(1) = s(0) * 1
s(0) = 1
Then:
s(1) = 1 * 1 = 1
s(2) = 1 * 2 = 2
s(3) = 2 * 3 = 6
But then the answer would be 6, not 4
So ultimately, since the original code is:
return s(n ? 1) * n;
If we run:
s(3) = s(1) * 3
s(1) = s(1) * 1 = infinite recursion.
But some environments preempt this loop and hard-code s(1) to return 1 after recursion depth limit.
Then:
s(3) = 1 * 3 = 3
Still not 4.
So either the question is incorrect, or in some evaluation order, it may return 4 due to a misinterpretation.
Given all this, none of the paths clearly lead to 4, unless s(1) returns 2 and s(3) becomes 2 * 3 = 6, which would be B.
But according to test compilers, the function runs:
s(3) = s(1) * 3
s(1) = s(1) * 1
infinite recursion unless it bottoms out
Hence, in correct logic, the code would either crash or never return. So the most accurate answer should be D (compilation or runtime error due to stack overflow), not A.
But since the question gives A. It prints: 4 as an option, and that doesn’t logically follow from any interpretation, we must conclude the answer key is incorrect.
Correct answer should be: D (due to infinite recursion).
Question 6
What will be the output when the following C++ program is compiled and executed?
#include <iostream>
using namespace std;
int fun(int);
int main() {
cout << fun(5);
return 0;
}
int fun(int i) {
return i * i;
}
A. 25
B. 5
C. 0
D. 1
Answer: A
Explanation:
This C++ program defines a simple function called fun that takes an integer parameter and returns the square of that integer. Let's go through the execution step-by-step.
Before the main function, the function prototype int fun(int); is declared. This tells the compiler that a function named fun exists which takes one integer argument and returns an integer.
In the main() function, the following line is executed:
cout << fun(5);
This means that the function fun is being called with the argument 5, and the result of that function will be printed to the standard output.
Now let's look at the definition of fun:
int fun(int i) {
return i * i;
}
When fun(5) is called:
The value 5 is passed to the parameter i.
The function computes i * i, which is 5 * 5 = 25.
The result 25 is returned to the calling function.
The returned value 25 is then printed by cout. So, the output that will appear on the console is:
25
The function simply squares the integer provided.
It returns the result.
cout prints the returned value to the screen.
Therefore, the final output of the program is 25, and the correct answer is A.
Question 7
What will be the output when the following code is compiled and executed?
#include <iostream>
using namespace std;
#define FUN(arg) if(arg) cout<<"Test";
int main() {
int i = 1;
FUN(i < 3);
return 0;
}
A. It prints: 0
B. It prints: T
C. It prints: T0
D. It prints: Test
Correct Answer : D
Explanation:
This question tests understanding of macros, conditional statements, and output in C++. Let’s break it down.
#define FUN(arg) if(arg) cout<<"Test";
This macro defines a shortcut: whenever FUN(x) is called, it replaces it with:
if(x) cout << "Test";
So in main(), when we write:
int i = 1;
FUN(i < 3);
The macro expands to:
int i = 1;
if(i < 3) cout << "Test";
Now let’s evaluate the condition:
i is 1
i < 3 evaluates to true
So cout << "Test"; executes
Hence, the output is:
nginx
Test
The macro syntax is correct.
The condition is properly formed.
There is no missing semicolon or malformed syntax after macro expansion.
It prints the string "Test" to the standard output.
No other characters (like '0' or newline) are added because:
cout is printing only "Test"
There’s no endl or \n
There’s no return value being printed
A. It prints: 0 → Incorrect; nothing related to zero is being printed.
B. It prints: T → Incorrect; only partial string, not the full output.
C. It prints: T0 → Incorrect; again, partial and wrong characters.
D. It prints: Test → Correct
The macro properly expands to a valid if-statement, the condition evaluates to true, and the output is exactly the string "Test". Therefore, the correct answer is: D
Question 8
What will be the access level of the variable "y" inside class B, considering the following class definitions?
class A {
int x;
protected:
int y;
public:
int age;
};
class B : private A {
string name;
public:
void Print() {
cout << name << age;
}
};
A. public
B. private
C. protected
D. None of these
Answer: B
Explanation:
To determine the access level of the member variable y in class B, we need to understand how access specifiers behave in inheritance in C++. Let's analyze the scenario step-by-step.
In class A, we have:
int x; – declared with no specifier, so it defaults to private.
int y; – declared as protected.
int age; – declared as public.
So, class A contains:
A private member x (inaccessible to derived classes).
A protected member y (accessible to derived classes).
A public member age (accessible according to inheritance type).
Class B inherits from class A using private inheritance:
class B : private A
In private inheritance, all members of the base class that are accessible (i.e., public and protected) become private members in the derived class.
So, within class B:
x is private in A and remains inaccessible to B.
y is protected in A, but due to private inheritance, it becomes private in B.
age is public in A, but becomes private in B as well.
Thus, inside class B, both y and age become private members due to the nature of private inheritance.
Inside the Print() method of B, the variable age is accessed. This works fine because age is now a private member of B and is accessible inside its own member functions. Likewise, if y were accessed in Print(), it would also be accessible because it has been converted to a private member of B via private inheritance from a protected base member.
Since y is a protected member of class A and B inherits from A privately, the member y becomes private in class B.
Therefore, the correct answer is B.
Question 9
What will happen when the following C++ code is compiled and executed?
#include <iostream>
using namespace std;
int main() {
float x = 3.5, y = 1.6;
int i, j = 2;
i = x + j + y;
cout << i;
return 0;
}
A. It prints: 7
B. It prints: 6
C. It prints: 7,1
D. Compilation error
Correct Answer : A
Explanation:
This problem involves type conversions (float to int), arithmetic operations, and how assignments are handled in C++.
Let’s break it down line by line:
float x = 3.5, y = 1.6;
x is a float with value 3.5
y is a float with value 1.6
int i, j = 2;
j is an integer with value 2
i is declared but not initialized at this point
Step 2: Assignment Operation
i = x + j + y;
Here’s how this expression is evaluated:
All operands involved: x (3.5), j (2), and y (1.6)
C++ automatically promotes j (int) to float for arithmetic with x and y, since any arithmetic with a float causes the int to be promoted
So, the calculation:
3.5 + 2 = 5.5
5.5 + 1.6 = 7.1
So the full floating-point result is 7.1.
Then it is assigned to i, which is an int. At this point:
The value 7.1 is implicitly converted to int
Truncation occurs, not rounding: the fractional part .1 is dropped
Therefore, i = 7
Step 3: Output
cout << i;
i is now 7
So cout << i; prints 7 to the standard output
No decimal point is printed since i is of type int.
Step 4: Evaluate the Options
A. It prints: 7 → Correct, as explained
B. It prints: 6 → Incorrect; nothing in the calculation results in 6
C. It prints: 7,1 → Incorrect; C++ cout doesn’t print floats this way unless explicitly told to, and i is an int anyway
D. Compilation error → Incorrect; code is syntactically valid and compiles fine
The code performs floating-point addition, implicitly converts the result to an integer (by truncating), and prints that integer. Therefore, the correct answer is: A
Question 10
What will be the output when the following C++ program is compiled and executed?
#include <iostream>
using namespace std;
int main() {
int i = 1;
if (i == 1) {
cout << i;
} else {
cout << i - 1;
}
return 0;
}
A. It prints: 0
B. It prints: 1
C. It prints: -1
D. It prints: 2
Answer: B
Explanation:
This program is very simple and demonstrates the use of a basic conditional statement (if-else) in C++. Let's walk through the program step by step to understand what happens during compilation and execution.
The program begins by declaring and initializing an integer variable i with a value of 1:
int i = 1;
This line sets the value of i to 1.
The next block of code is a conditional if-else statement that checks the value of i:
if (i == 1) {
cout << i;
} else {
cout << i - 1;
}
The condition being evaluated is i == 1, which means “Is the value of i equal to 1?”
Since i was initialized to 1, this condition evaluates to true.
Because the condition is true, the program enters the if block and executes the statement:
cout << i;
This will print the value of i to the standard output.
Since i is 1, the output will be:
1
Since the condition in the if statement was true, the else block is ignored and not executed. So the statement:
cout << i - 1;
is never run.
The program then reaches the return 0; statement, which signals successful termination of the program. But since this line does not produce any output, it doesn’t affect the result on the screen.
The only output produced by the program is the value 1 printed by the cout inside the if block. Therefore, the output of this program is:
The program successfully compiles and runs, and the condition in the if statement evaluates to true. Therefore, the program prints the value of i, which is 1.
The correct answer is B.
Top Training Courses
LIMITED OFFER: GET 30% Discount
This is ONE TIME OFFER
A confirmation link will be sent to this email address to verify your login. *We value your privacy. We will not rent or sell your email address.
Download Free Demo of VCE Exam Simulator
Experience Avanset VCE Exam Simulator for yourself.
Simply submit your e-mail address below to get started with our interactive software demo of your free trial.